Monday, November 25, 2024
Building My First Blog: Components, MDX, and React Excellence
Posted by
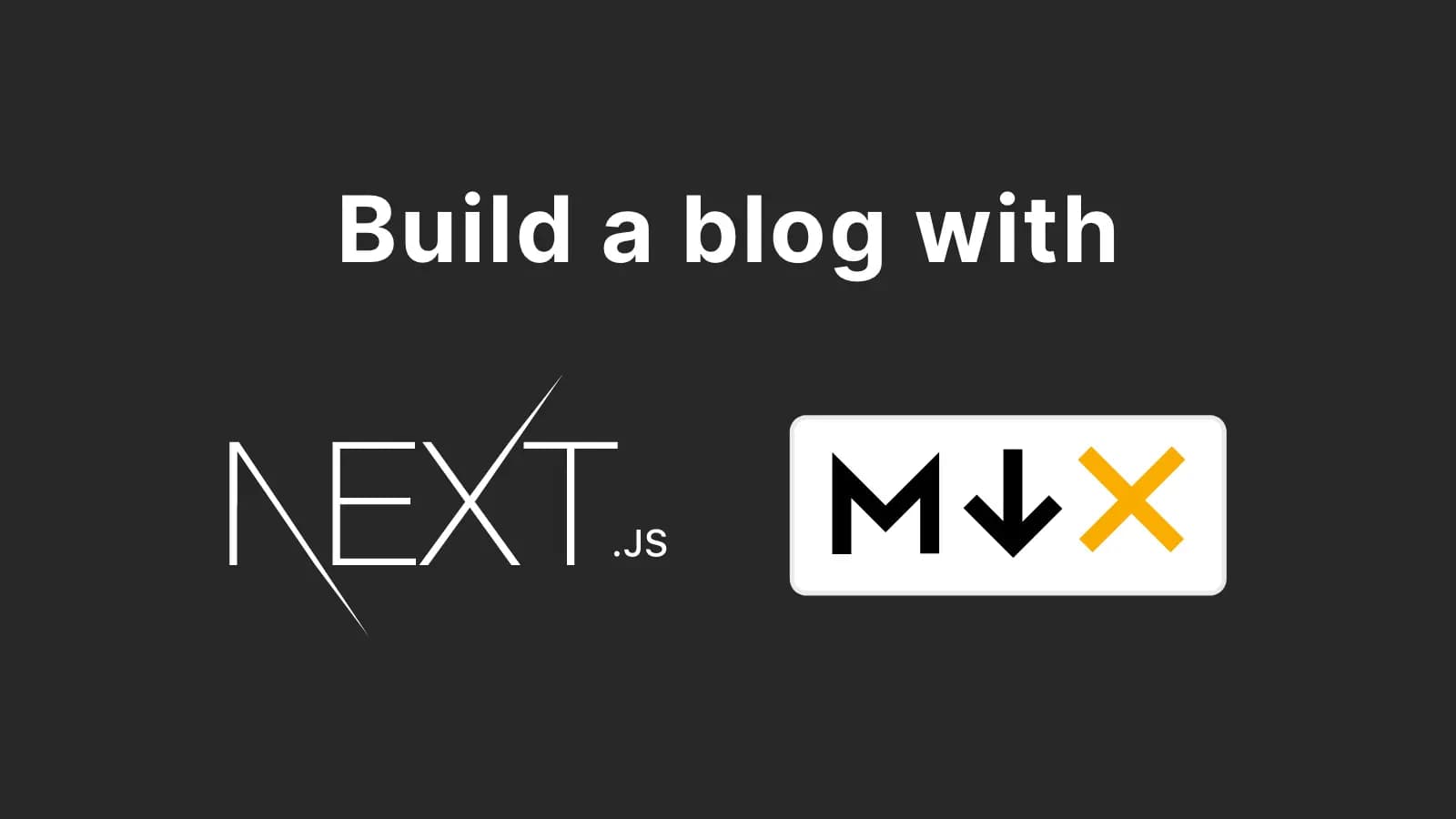
Stepper
This section previews the stepper component.
In this guide, we utilize a custom Stepper
component, specifically designed for AriaDocs, which enables users to display step-by-step instructions directly within the markdown render.
Stepper Preview
Step 1: Clone the AriaDocs Repository
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec interdum, felis sed efficitur tincidunt, justo nulla viverra enim, et maximus nunc dolor in lorem.
Step 2: Access the Project Directory
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Proin non neque ut eros auctor accumsan. Mauris a nisl vitae magna ultricies aliquam.
Step 3: Install Required Dependencies
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Pellentesque ut ipsum nec nulla ultricies porttitor et non justo.
Stepper Code
<Stepper>
<StepperItem title="Step 1: Clone the AriaDocs Repository">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec interdum,
felis sed efficitur tincidunt, justo nulla viverra enim, et maximus nunc
dolor in lorem.
</StepperItem>
<StepperItem title="Step 2: Access the Project Directory">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Proin non neque ut
eros auctor accumsan. Mauris a nisl vitae magna ultricies aliquam.
</StepperItem>
<StepperItem title="Step 3: Install Required Dependencies">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Pellentesque ut
ipsum nec nulla ultricies porttitor et non justo.
</StepperItem>
</Stepper>
Tabs
The Tabs
component allows you to organize content into multiple sections, enabling users to switch between them easily. This is particularly useful for displaying related content in a compact manner.
Tabs Preview
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Tabs Props
Prop | Type | Default | Description |
---|---|---|---|
defaultValue | string | null | The value of the tab that is selected by default. |
className | string | "" | Additional CSS classes for styling the Tabs component. |
Tabs Code
<Tabs defaultValue="java" className="pt-5 pb-1">
<TabsList>
<TabsTrigger value="java">Java</TabsTrigger>
<TabsTrigger value="typescript">TypeScript</TabsTrigger>
</TabsList>
<TabsContent value="java">
```java
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```</TabsContent>
<TabsContent value="typescript">
```typescript
// helloWorld.ts
function helloWorld(): void {
console.log("Hello, World!");
}
helloWorld();
```</TabsContent>
</Tabs>
Notes
The Note
component allows you to display different types of messages such as general notes, warnings, or success notifications. Each type is styled accordingly, providing a clear visual cue to the user.
Notes Preview
Note:
This is a general note to convey information to the user.
Danger:
This is a danger alert to notify the user of a critical issue.
Warning:
This is a warning alert for issues that require attention.
Success:
This is a success message to inform the user of successful actions.
Notes Props
Prop | Type | Default | Description |
---|---|---|---|
title | string | "Note" | Sets the title of the note. |
type | "note" , "danger" , "warning" , "success" | "note" | Determines the visual style of the note. |
Notes Code
<Note type="note" title="Note">
This is a general note to convey information to the user.
</Note>
<Note type="danger" title="Danger">
This is a danger alert to notify the user of a critical issue.
</Note>
<Note type="warning" title="Warning">
This is a warning alert for issues that require attention.
</Note>
<Note type="success" title="Success">
This is a success message to inform the user of successful actions.
</Note>
Code Block
The Code Block in this documentation allows you to display code snippets with optional line numbering and line highlighting.
Code Block Preview
function isRocketAboutToCrash() {
// Check if the rocket is stable
if (!isStable()) {
NoCrash(); // Prevent the crash
}
}
In this example, line numbers are displayed for lines 1 to 4. You can specify which lines to highlight using the format {2,3-5}
.
Code Block Usage
You can directly use the following syntax to create a code block with line numbers and highlight specific lines:
```javascript:main.js showLineNumbers {3-4}
function isRocketAboutToCrash() {
// Check if the rocket is stable
if (!isStable()) {
NoCrash(); // Prevent the crash
}
}
```
Code Block Features
- Line Numbers: Enable line numbers by adding
showLineNumbers
after the opening backticks. - Highlight Lines: Specify lines to highlight using curly braces (e.g.,
{2,3-5}
). - Syntax Highlighting: Use the appropriate language for syntax highlighting.
Image and Link
In AriaDocs, all links and images written in Markdown are automatically converted to their respective Next.js components. This allows for better optimization and performance in your application.
Links
When you create a link in your Markdown, it is converted to the Next.js Link
component. This enables client-side navigation and improves loading times. Here’s an example of how a Markdown link is transformed:
Links Markdown
[Visit OpenAI](https://www.openai.com)
Links Rendered Output
The above Markdown is converted to:
<Link href="https://www.openai.com" target="_blank" rel="noopener noreferrer">
Visit OpenAI
</Link>
Images
Similarly, images in Markdown are transformed into the Next.js Image
component. This allows for automatic image optimization, such as lazy loading and resizing, which enhances performance and user experience. Here’s an example:
Images Markdown
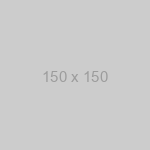
Images Rendered Output
The above Markdown is converted to:
<Image
src="https://via.placeholder.com/150"
alt="Alt text for the image"
width={800}
height={350}
/>
Benefits
- Performance Optimization: Automatic conversion to Next.js components ensures optimized loading of images and links.
- Improved User Experience: Client-side navigation with Next.js
Link
improves the browsing experience. - Responsive Images: Next.js
Image
component handles responsive images, providing the best quality for various device sizes.
By utilizing these features, you can ensure that your documentation is not only visually appealing but also performs efficiently.
Custom
To add custom components in AriaDocs, follow these steps:
-
Create Your Component: First, create your custom component in the
@components/markdown
folder. For example, you might create a file namedOutlet.tsx
. -
Import Your Component: Next, open the
@lib/markdown.ts
file. This is where you'll register your custom component for use in Markdown. -
Add Your Component to the Components Object: In the
@lib/markdown.ts
file, import your custom component and add it to thecomponents
object. Here’s how to do it:
import Outlet from "@/components/markdown/outlet";
// Add custom components
const components = {
Outlet,
};
- Using Your Custom Component in Markdown: After registering your component, you can now use it anywhere in your Markdown content. For instance, if your
Outlet
component is designed to display additional information, you can use it as follows:
Markdown Example
<Outlet>
This is some custom content rendered by the Outlet component!
</Outlet>
Custom Rendered Output
This will render the content inside the Outlet
component, allowing you to create reusable and dynamic Markdown content.
By following these steps, you can extend the capabilities of your Markdown documentation and create a more engaging user experience.
🚀📁